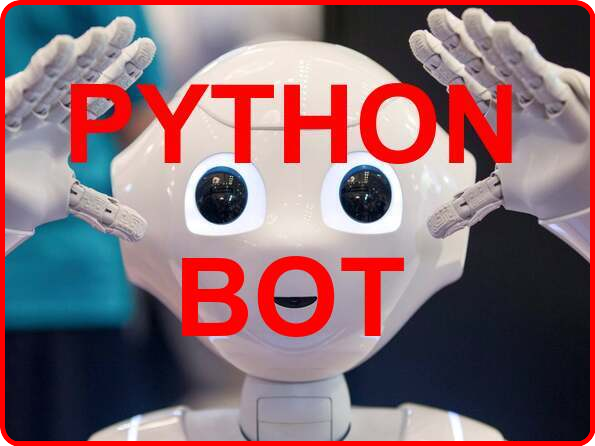
This tutorial provides a simple Python scripting guide for creating a bot in Runescape. While some may refer to it as an autoclicker rather than a bot, the terms are interchangeable. No prior knowledge of Python is necessary as this tutorial is beginner-friendly. The Python script itself is straightforward, consisting of less than 10 lines of code that enable task automation in Runescape. However, it’s important to temper your expectations regarding the capabilities of this method.
Runescape offers numerous skills that require minimal movement, making this simple Python bot particularly effective for skills such as cooking, smithing, thieving, fletching, mining, and other AFK (Away From Keyboard) skills. While you have the option to make the script more complex, it’s advisable to start with a simple version to avoid making too many mistakes.
Starting out, it’s common to make errors. This simple Python bot for Runescape carries the risk of getting you banned, and only experience can help you navigate this issue. With time, you’ll learn which skills can be bot-controlled without attracting penalties. However, it’s crucial to note that just because a skill is highly AFK doesn’t mean it should be automated. Therefore, I strongly recommend against using this method on your main account.
Before embarking on creating your own Runescape bot using Python, it’s wise to utilize a new account that you don’t mind losing. Additionally, employing a VPN (Virtual Private Network) is recommended to avoid being chain banned by Runescape. While this method is compatible with both Old School Runescape and Runescape 3, the latter is more suitable due to its highly AFK nature. Hence, starting with Runescape 3 is advised.
It’s worth mentioning that this script is not intended for bot farmers and works best with a single account. While some individuals may use it for two accounts, attempting to run it with more accounts will yield suboptimal results. This simple Runescape Bot Script is designed to assist players who have limited time for grinding, those looking to earn money for a bond, and anyone seeking to upgrade their gear or maximize AFK skills.
It’s important to note that the majority of players in the Runescape Community are against botting and macroing. You can find numerous videos on YouTube highlighting this sentiment. Additionally, Jadex, the anti-cheating system, will ban you for botting. I won’t advise you on which AFK skills to avoid botting, as the best way to evade bans is simply not to bot. These scripts can easily get you into trouble, and even running the script for a little over two hours or performing 1,000 clicks in less than a minute on the same spot can lead to a ban.
Don’t forget to utilize a VPN and create a few throwaway accounts. In my experience, new “botters” are prone to making mistakes and getting caught. Conduct your own research on Google to find the best and worst spots for botting. You should be able to find examples of Python bots for Runescape on GitHub. However, it’s advisable not to run these scripts; instead, use them as a learning resource to understand what modules and methods cheaters use to disrupt the game for others.
To begin creating your own Python autoclicker or bot script, you’ll need a version of Python that is 3.5 or higher and the pyautogui module. This module enables clicking on the screen and can also detect images. You can find the pyautogui documentation at this link.
Let’s delve into the script:
import pyautogui
x = pyautogui.position()
print(x)
Line 1: Import the pyautogui module in Python to utilize its built-in functions.
Line 2: Create a variable named x
(you can choose any name following Python variable naming conventions).
Assign the function pyautogui.position()
to x
. This function returns the X and Y coordinates of the mouse cursor, which is extremely useful. Before clicking anywhere on the screen, the script needs to know where to click. This function provides the necessary X and Y coordinates. Saving the values of x
and y
allows their usage in other functions.
Upon running the script, the output will resemble the following:
Point(x=538, y=304)
How to Use the X and Y Position Provided by pyautogui.position()
to Click on the Screen:
To click on the screen at a specific X and Y position, use the pyautogui.click(x, y)
function.
import pyautogui
x = 538
y = 304
z = pyautogui.click(x, y) # or z = pyautogui.click(538, 304)
print(z)
This short code will move the mouse to the specified X and Y location on the screen and perform a left-click.
Time Sleep Function:
To instruct your script to wait before clicking elsewhere, you can utilize the time
module in Python, specifically time.sleep(seconds)
.
import pyautogui
import time
pyautogui.click(538, 304)
time.sleep(20) # Wait for 20 seconds before clicking elsewhere
pyautogui.click(1124, 824)
As you can see, there are no variables used in this example; the code gets straight to the task.
Random Function:
By incorporating the random
Python module, you can introduce a random waiting period between 20 to 25 seconds. The random
module generates a random number within the specified range each time the script is run. To achieve this, use random.randint(20, 25)
within the time.sleep()
function. Here’s an example: time.sleep(random.randint(20, 25))
.
import pyautogui
import time
import random
pyautogui.click(538, 304)
time.sleep(random.randint(20, 25))
pyautogui.click(1124, 824)
Looping Your Python Script Forever or for a Desired Duration:
Here are a few examples:
The first code example will execute the program 12 times:
x = 0
while x < 12:
pyautogui.click(1154, 442)
time.sleep(50)
x += 1
The second code example will run indefinitely until you terminate the program:
while True:
pyautogui.click(1534, 596)
time.sleep(int(random.randint(4, 7)))
This covers the basics of creating a simple bot or autoclicker using Python. For further understanding of pyautogui, refer to the documentation linked here.
Please note that this blog post is still a work in progress. I will continue to improve the article and add more examples in the future.